Runtime Stack Mechanism in Java
For every thread, JVM creates a separate runtime stack. The run time stack has one main thread and corresponding methods called by that main thread. Every entry in a stack is called stack frame or activation records.
CASE I: If the method doesn't have any exceptions(Normal Flow of Execution)
Demo Example:
package com.navneet.javatutorial;
package com.navneet.javatutorial;
public class DefaultExceptionHandling {
public static void main(String[] args) {
m1();
}public static void m1() {
m2();
System.out.println("This is method m1");}
public static void m2() {
System.out.println("This is method m2");}
}
In the above example, JVM creates three stack frame one for the main thread, second for method m1 and third for method m2. The below figure describes the Runtime stack Creation architecture.
Creation of runtime stack frame by JVM
Destruction of runtime stack:
1-If the method m2 completed successfully without any exception control goes to the m1 method and corresponding entry from the stack is deleted and this process continues until the main method and also deleted the empty runtime stack by JVM at the end.
CASE II: If the method have exceptions(Abnormal Flow of Execution)
Creation of runtime stack frame by JVM
The below example has ArithmeticException at method m2() location the JVM will check any exception handling code is there if not there method m2 will be responsible to create exception object because of exception is raised on method m2 and corresponding entry from the stack will be deleted and control goes to method m1().
JVM again check the caller method have any exception handling code are not if not JVM terminates the method abnormally and deleted the corresponding entry from the stack.
The above process continues until the main thread. If the main thread(main method) does?t have any exception handling code the JVM also terminates the main method abnormally and the default exception handler is responsible to print the exception message to the console which is the part of JVM. We will discuss the default exception handler in the next tutorial.
Destruction of runtime stack:
This program describes the abnormal termination/Execution.
Demo Example:
package com.navneet.javatutorial;public class RuntimeStackDemo {
public static void main(String[] args) {
m1();
}
public static void m1(){
m2();
System.out.println("This is method m1");
}
public static void m2(){
System.out.println(100/0);
System.out.println("This is method m2");}
}
Console O/P:
Exception in thread "main" java.lang.ArithmeticException: / by zero
at com.navneet.javatutorial.RuntimeStackDemo.m2(RuntimeStackDemo.java:13)
at com.navneet.javatutorial.RuntimeStackDemo.m1(RuntimeStackDemo.java:9)
at com.navneet.javatutorial.RuntimeStackDemo.main(RuntimeStackDemo.java:6)
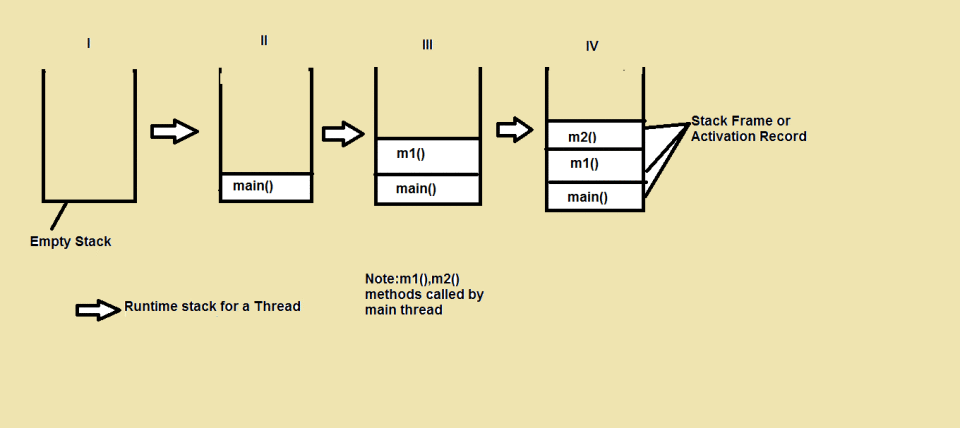
Fig.3
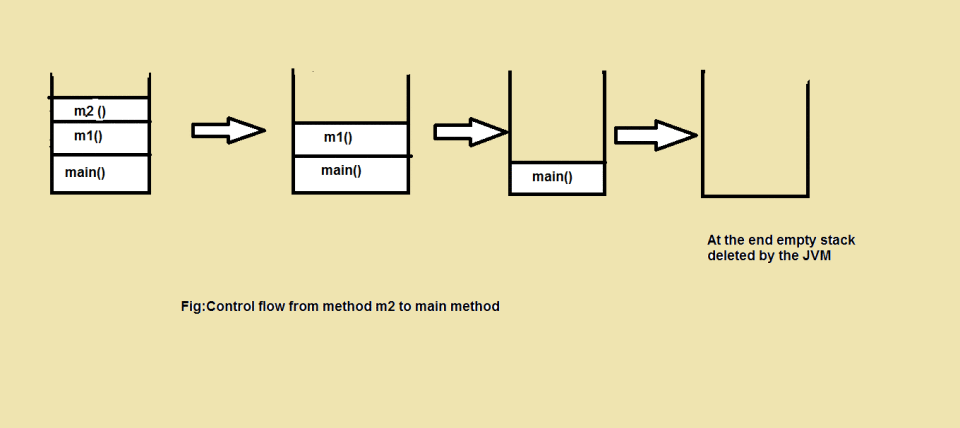