Flow control in try-catch-finally blocks
The code that might throw certain exceptions must be enclosed by either of the following two ways.
1-try is a block that catches the exception.The try is a container (handler) for exceptions.The try block must be followed by catch and finally block.
2-Add throws clause that specifies the exception.The method must provide a throws clause that lists the exceptions handled by that method.The code structure are as below format.
public static void m1()throws ArithmeticException {
System.out.println(10 / 0);
System.out.println("Hello world");
}
Catching and Handling Exceptions:
Java provides three most important exception handling components- try, catch and finally blocks to handle exceptions.
1-The try Block
The main purpose of try block is to handle exceptional or risky code.The first step to set up exception handle in java is to enclose the code which thows exception within try block ,a try block looks like the following:
2-The catch Blocks
The catch block catches the exceptions throwing by try block.If the try block contains multiple type exceptions so corresponding matched catch block executed.No code can be between the end of the try block and the beginning of the first catch block.The catch block contains code that are executed if any exceptions are invoking by try block.Catching More Than One Type of Exception with one catch block
Important Note:
In Java 7 and later version, a single catch block can handle more than one type of exception.This feature can reduce code copying and less code to write.
Structure of new modified catch block
In the new modified catch clause each exception type separated by vertical bar |
3-Java finally block
The finally block always executes when the try block exits.The main purpose of finally block is to do resource clean up and close activity.
Demo Program:
package com.navneet.javatutorial;
public class MultipleCatchBlock {
public static void main(String[] args) {
try {
System.out.println("This is statement 1");
System.out.println("This is statement 2" + 10 / 0);
System.out.println("This is statement 3");
}catch (ArithmeticException e) {
System.out.println("task1 is completed");
}finally {
System.out.println("This is statement 4");
}System.out.println("This is statement 5");
}}
Flow control in try-catch-finally blocks:
CASE I: If no exception occurs in try block(Normal flow of execution)
If no exception occurs inside try block the corresponding catch block is not executed and control goes to finally block.The below example describes the program execution flow clearly.
Demo Example:
package com.navneet.javatutorial;public class MultipleCatchBlock {
public static void main(String[] args) {
try {
System.out.println("Statement 1");System.out.println("Statement 2");
}catch (ArithmeticException e) {
System.out.println("Statement 3 ");
}finally {
System.out.println("Statement 4");
}
}}
CASE II: If exception occurs in try block (Exceptional flow of execution)
If the try block have any exceptional statement the corresponding matched catch block executed and the control goes to finally block.The demo program describes the execution flow.
Demo Example:
Fig.2package com.navneet.javatutorial;
public class MultipleCatchBlock {
public static void main(String[] args) {
try {
System.out.println("Statement 1");
System.out.println( 10/ 0);}
catch (ArithmeticException e) {
System.out.println("Statement 3");
}finally {
System.out.println("Statement 4");
}
}}
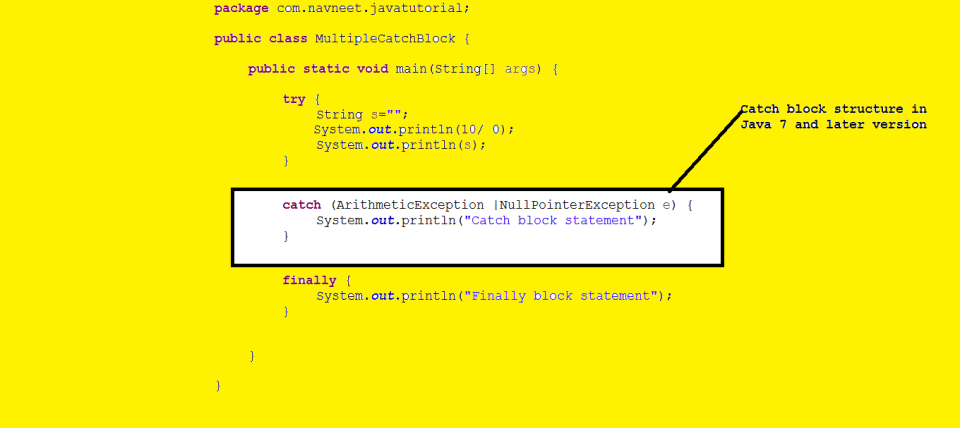
Fig.3
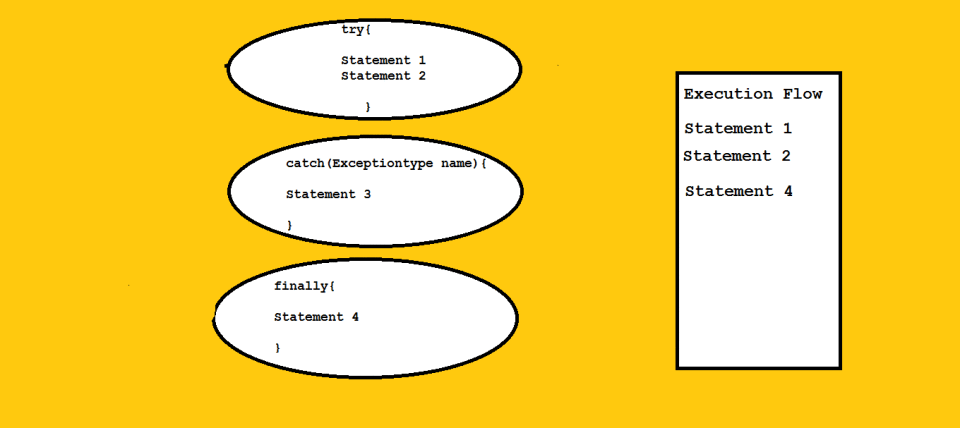
Fig.4
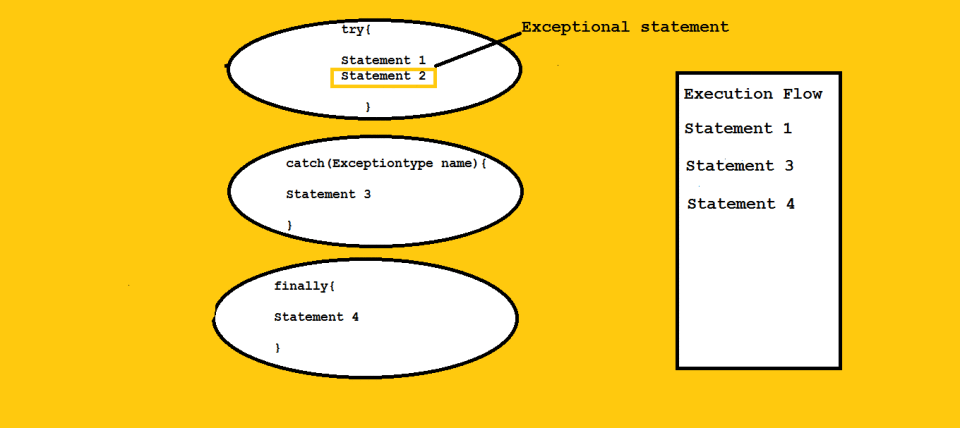